자바 백준 13420번
브론즈 2
https://www.acmicpc.net/problem/13420
13420번: 사칙연산
사칙연산은 덧셈, 뺄셈, 곱셈, 나눗셈으로 이루어져 있으며, 컴퓨터 프로그램에서 이를 표현하는 기호는 +, -, *, / 와 같다. 아래는 컴퓨터 프로그램에서 표현한 사칙 연산의 예제이다. 3 * 2 = 6 문
www.acmicpc.net
문제 보기
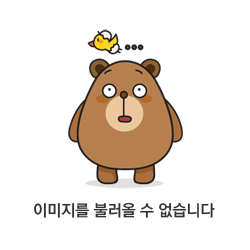
분류: 수학, 구현, 문자열, 사칙연산
코드 풀이
import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int T = sc.nextInt(); sc.nextLine(); for (int i = 0; i < T; i++) { String[] input = sc.nextLine().split(" "); long operand1 = Long.parseLong(input[0]); char operator = input[1].charAt(0); long operand2 = Long.parseLong(input[2]); long answer = Long.parseLong(input[4]); long result = Calculator.calculate(operand1, operator, operand2); if (result == answer) { System.out.println("correct"); } else { System.out.println("wrong answer"); } } } } class Calculator { public static long calculate(long operand1, char operator, long operand2) { long result; switch (operator) { case '+': result = operand1 + operand2; break; case '-': result = operand1 - operand2; break; case '*': result = operand1 * operand2; break; case '/': result = operand1 / operand2; break; default: result = 0; } return result; } }
'공부하기 > 백준' 카테고리의 다른 글
[Java] 백준 풀기 1547 - 공 (0) | 2024.03.08 |
---|---|
[Java] 백준 풀기 11645 - I’ve Been Everywhere, Man (0) | 2024.03.07 |
[Java] 백준 풀기 26068 - 치킨댄스를 추는 곰곰이를 본 임스 2 (0) | 2024.03.05 |
[Java] 백준 풀기 9536 - 여우는 어떻게 울지? (0) | 2024.03.04 |
[Java] 백준 풀기 9935 - 문자열 폭발 (2) | 2024.03.03 |